On this page
For Android developers, understanding the concepts of Deep links and App Links is crucial to providing a seamless user experience for end users. This guide delves into the differences between Deep links and App Links, importance of the security concerns, and how to implement and test these links effectively.
Whether you're a developer integrating Web3Auth’s Android SDK or working with ReactNative/Flutter, this guide will help you master the art of handling links easily in your Android applications.
Understanding Deep links and App Links
What are deep links?
To start off, let us define what Deep links are. They are strings of characters that enable users to access specific content or features within an Android app. They have three essential components — scheme, authority, and path.
These links can handle custom schemes or universal resource identifiers (URIs) for seamless navigation.
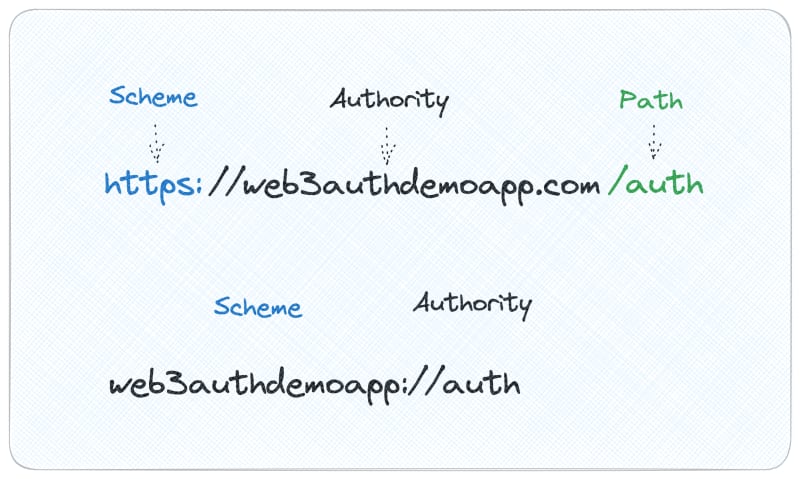
What are App Links?
App Links are a specialized form of Deep links that allow Android apps to handle HTTP and HTTPS web links. With App Links, developers can associate their app with specific web domains, providing users with a seamless experience when navigating between websites and the app.
Difference between Android App Links and Web links
While Android App Links and Web links share similarities in their functionality as methods to link users to specific content, they have fundamental differences that cater to different user scenarios. Understanding these distinctions will help developers make informed decisions about which link type to use in various situations.
Android App Links
Android App Links are a specialized form of deep links that offer a more seamless and integrated user experience for Android users. When a user clicks on an Android App Link, it automatically opens the associated app if it is installed on the device. This behavior eliminates the need for a disambiguation dialog which asks users to choose between opening the link in the app or the browser.
Key characteristics of Android App Links:
1. Immediate app invocation — Android App Links directly invoke the app, providing users with a faster and smoother experience as they are taken directly to the relevant content within the app.
2. Verified association — Android App Links are explicitly associated with a specific app through a verification process, which ensures that only the designated app can handle these links. This verification is achieved through the use of Digital Asset Links JSON files hosted on the website's domain.
3. No disambiguation dialog — The absence of a disambiguation dialog streamlines the user journey and reduces the risk of users inadvertently choosing the wrong app to handle the link.
Web links
On the other hand, web links are links that use the HTTP and HTTPS schemes and open in the device's default web browser. They are more generic and platform-agnostic, providing a consistent experience across different operating systems and devices.
Key characteristics of Web links:
1. Universal accessibility — Web links are accessible to all users, regardless of whether they have the app installed on their device. When users click on a web link, it opens the associated web page in the browser, making it a useful method for sharing content with a broader audience.
2. Not app-specific — Unlike Android App Links, web links do not require any specific app association or verification. They can be used universally and do not rely on a particular app's presence on the device.
3. Potential disambiguation — Since Web links are not bound to a specific app, users may have multiple apps that can handle a particular link. A disambiguation dialog may appear in such cases, prompting users to select the desired app for link handling.
Ensuring App Link handling in your Android app
Implementing Deep links
Deep links allow users to directly access specific content within your app directly, enhancing user engagement and navigation. To ensure your Android app can handle incoming deep links, follow these crucial steps:
Step 1 — Add intent filters for incoming links to your app's manifest file
In your app's AndroidManifest.xml file, you need to declare intent filters that define which links your app can handle. These filters specify the actions and data schemes that your app can respond to. Here's an example of how to add an intent filter for your app's Deep link:
```xml
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Accept URIs: {YOUR_APP_PACKAGE_NAME}://* -->
<data android:scheme="YOUR_APP_PACKAGE_NAME" />
</intent-filter>
```
In this example, the intent filter listens for "android.intent.action.VIEW" actions and includes "android.intent.category.DEFAULT" and "android.intent.category.BROWSABLE" categories. These categories ensure the link can be opened from a web browser or another app. Replace "YOUR_APP_PACKAGE_NAME" with your actual app's package name. This scheme, along with the wildcard "*", allows your app to handle any deep link associated with your app's package name.
Step 2 — Read data from incoming intents to determine the appropriate content to render
When a user clicks a Deep link or an app programmatically invokes a URI intent, your app receives the deep link as an Intent. To handle the Deep link appropriately, you must read the data provided by the incoming Intent to determine the content to render. This is typically done in the activity that is launched by the Deep link.
In your activity's `onCreate` method, you can retrieve the data from the Intent like this:
```kotlin
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.main)
val intentData: Uri? = intent?.data
// Use the intentData to determine the appropriate content to render
}
```
Additionally, to handle cases where the app is already active (in the background) when the deep link is triggered, you need to override the `onNewIntent` method:
```kotlin
override fun onNewIntent(intent: Intent?) {
super.onNewIntent(intent)
// Handle user signing in when app is active
web3Auth.setResultUrl(intent?.data)
}
```
In this example, `web3Auth.setResultUrl(intent?.data)` is a hypothetical function call to a custom function in your app that handles the deep link data. You should replace it with your actual implementation for appropriately handling the deep link data within your app.
With these steps, your app will be able to receive and handle deep links effectively, providing a seamless experience for users as they navigate directly to specific content within your Android application.
Security concerns with Deep links
Deep links, being a powerful way to navigate users directly to specific content within an app, introduce unique security considerations that developers must address to protect user data and ensure a safe user experience. Here are some key aspects to consider when dealing with the security implications of Deep links.
Handling sensitive information
Deep links may sometimes carry sensitive information in their URIs, such as user authentication tokens, personal identifiers, or sensitive data related to a particular user's account. To safeguard this information, it is crucial to avoid passing sensitive data directly in the link's URI. Instead, use secure and encrypted methods like OAuth tokens or access keys to authenticate users and authorize access to sensitive resources.
Opaque tokens for authorization
When using Deep links to handle access to secure resources, it is best practice to utilize opaque tokens rather than passing explicit data in the link's URI. Opaque tokens are unique, cryptographically secure identifiers that are used for authentication and authorization purposes. These tokens can be generated on the server-side and linked to a specific user or session. By utilizing opaque tokens, you can minimize the risk of unauthorized access, as the actual user-specific information remains hidden within the token and cannot be easily tampered with by malicious actors.
Token expiration and invalidation
To further enhance security, ensure that the opaque tokens have a limited validity period. This means setting an expiration time for the tokens, after which they become invalid and unusable. By implementing token expiration, you can reduce the window of opportunity for potential attackers to exploit captured or leaked tokens.
Secure transport and storage
Deep links should always be transmitted over secure channels, preferably using HTTPS, to prevent eavesdropping and man-in-the-middle attacks. Any sensitive data associated with the link should be stored securely on the server-side, following industry-standard encryption practices.
User consent and awareness
When a deep link triggers an action that may have significant consequences, such as navigating the user outside the app or performing critical operations, it is essential to inform the user about the potential outcomes. Implementing clear and explicit user consent mechanisms before executing such actions will ensure that users are aware of the consequences and have a chance to confirm or cancel the action.
Handling link interception
Users have the ability to customize link-handling behavior on their devices, which means that other apps or browsers may intercept deep links before they reach your app. As a result, ensure that your app can handle such scenarios gracefully and take appropriate measures to prevent unauthorized access or actions when external apps intercept a link.
Implementing Android App Links
Android App Links enable a more integrated and seamless user experience by automatically opening the associated app when users click on web links that are associated with the app. To implement Android App Links correctly, follow these additional steps:
Step 1 — Add the autoVerify attribute to intent filters
To enable the system to automatically verify that Android App Links are associated with your app, you need to add the "autoVerify" attribute to the intent filters in your app's AndroidManifest.xml file. This attribute indicates that your app's links are trusted and verified by the domain they originate from. The verification process occurs when users install the app, and it helps ensure that only your app can handle the designated links.
Here's how to add the "autoVerify" attribute to your intent filters:
```xml
<intent-filter android:autoVerify="true">
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<!-- Accept URIs with the "http" and "https" schemes -->
<data android:scheme="http" />
<data android:scheme="https" />
<!-- Include one or more domains that should be verified -->
<data android:host="your_website_domain.com" />
</intent-filter>
```
Replace "your_website_domain.com" with the actual domain of your website that you want to associate with your app's links. Using the "autoVerify" attribute will streamline the user experience as Android will automatically verify the association between your app and the website.
Step 2 — Declare the association between your app and website
To establish the association between your Android app and the website hosting the links, you need to create a Digital Asset Links JSON file and host it on your website's domain. This JSON file contains information about your app, such as its package name and SHA-256 certificate fingerprint. It serves as a declaration that the specified website and your app are related.
The JSON file should be hosted at the following location on your website:
```
https://your_website_domain.com/.well-known/assetlinks.json
```
The content of the Digital Asset Links JSON file may look like this:
```json
[
{
"relation": ["delegate_permission/common.handle_all_urls"],
"target": {
"namespace": "android_app",
"package_name": "com.example.yourapp",
"sha256_cert_fingerprints": [
"YOUR_SHA256_CERT_FINGERPRINT"
]
}
}
]
```
Replace "com.example.yourapp" with your app's package name, and "YOUR_SHA256_CERT_FINGERPRINT" with the SHA-256 certificate fingerprint of your app's signing key.
Step 3 — Verify Android App Links
After adding the "autoVerify" attribute to your intent filters and hosting the Digital Asset Links JSON file on your website, you need to verify that your Android App Links are set up correctly. The verification process ensures that your app is associated with the specified website and that the correct link-handling policies are in place.
To verify the Android App Links, you can use the following command using the Android Debug Bridge (adb) tool:
```
$ adb shell am start
-a android.intent.action.VIEW
-c android.intent.category.BROWSABLE
-d "https://your_website_domain.com"
```
Replace "https://your_website_domain.com" with an actual link associated with your app.
After performing these steps and successfully verifying the Android App Links, your app will offer a seamless user experience. Android will automatically open the app when users click links associated with your website. This streamlined experience enhances user engagement and encourages users to interact more effectively with your app's content.
How Web3Auth simplifies Deep links
Web3Auth is a powerful tool that simplifies implementing and managing deep links in Android apps. It provides developers a user-friendly and efficient solution, enabling them to handle DeepLinks/AppLinks seamlessly within their applications. Let's delve into more context about how Web3Auth simplifies the handling process:
Whitelisting links
Web3Auth's dashboard allows developers to easily whitelist specific links that should be handled by the app as deep links. Whitelisting ensures that only the specified links, which are essential for the app's functionality, will be processed as deep links. This streamlines the handling process, as the app will not be unnecessarily triggered for links that are not relevant to its core functionalities.
Setting result URLs
Web3Auth enables developers to set result URLs effortlessly. Result URLs are crucial for seamless user interactions when handling deep links. When the app finishes processing a deep link and performs specific actions based on the link's content, it can redirect users back to a designated result URL within the app. This ensures that users have a smooth experience even after interacting with external links that lead them back to the app.
User-friendly implementation
By utilizing Web3Auth's streamlined dashboard, developers can avoid the complexities of manually implementing deep links and handling various URI schemes. Web3Auth abstracts much of the technical details and provides a simplified interface, making it easier for developers to integrate deep link functionality into their Android apps without the need for extensive code modifications.
By leveraging the capabilities of Web3Auth's dashboard, developers can streamline the process of integrating and managing deep links in their Android apps. This user-friendly approach saves time and effort while providing a robust and reliable deep link handling solution.
As a result, developers can focus more on building engaging user experiences and less on the intricacies of link management, ultimately leading to a more efficient and user-centric app development process.
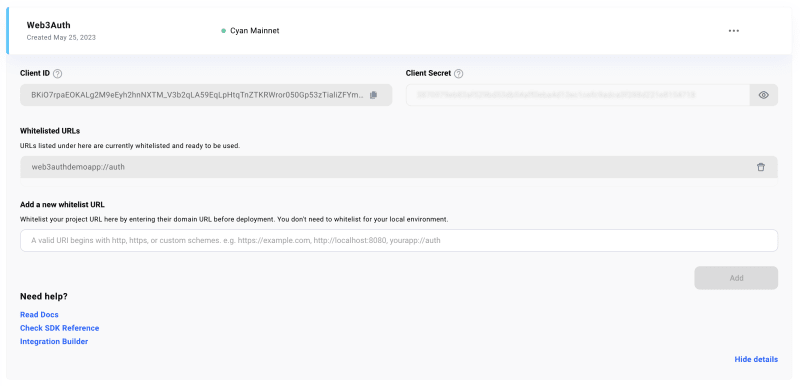
Conclusion
Deep links and App Links are essential tools for providing a seamless user experience in Android applications. Understanding the differences between these link types, implementing them correctly, and ensuring security measures are in place will enhance your app's functionality.
With the help of Web3Auth, developers can simplify the process of handling deep links and enhance the user experience in their Android apps.
So, go ahead and leverage the power of deep linking to take your users exactly where they want to go!